146. LRU 缓存 - 力扣(LeetCode)
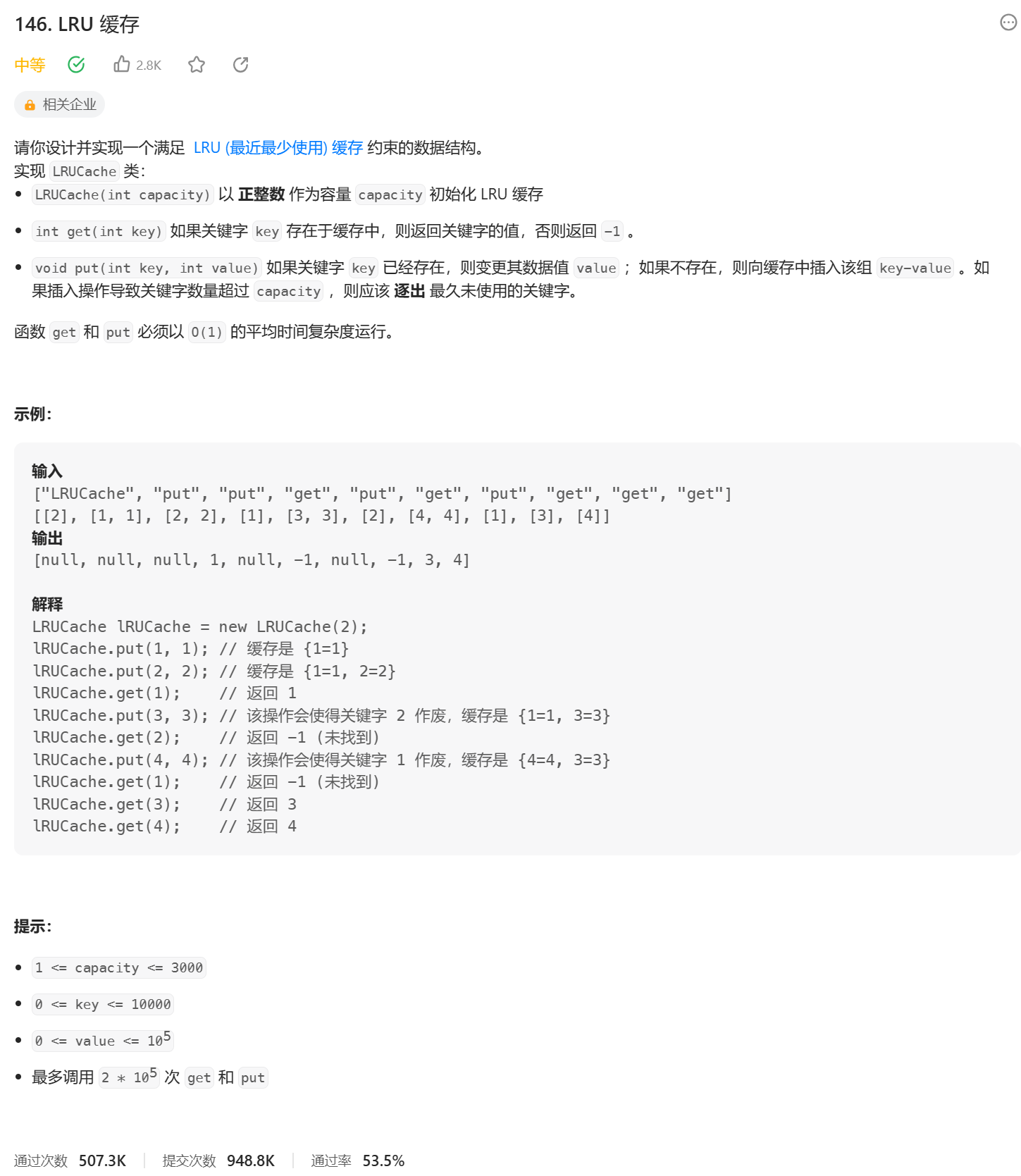
list Elemet 双向列表;
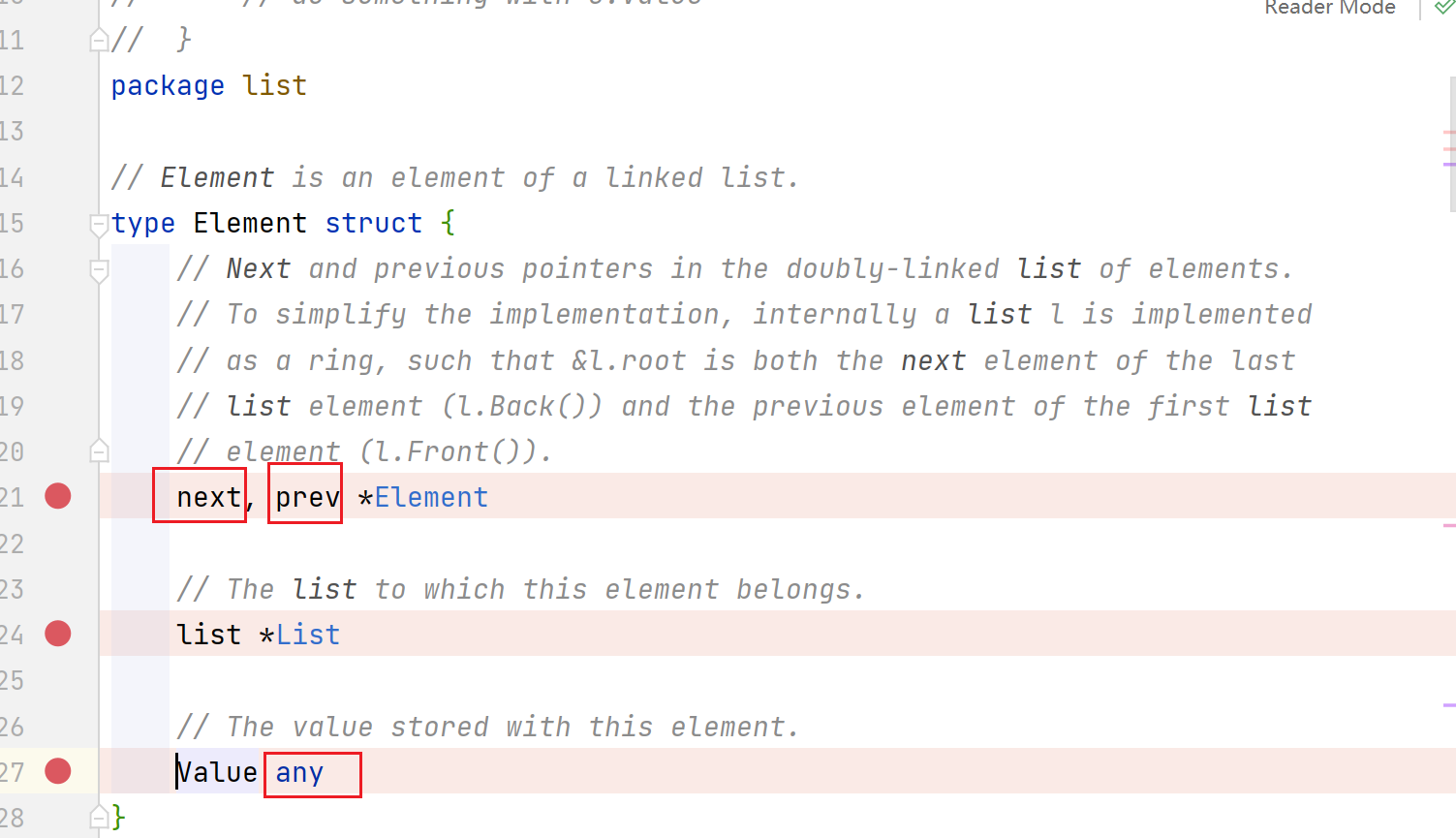
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| import "container/list"
type LRUCache struct { cap int cache map[int]*list.Element list *list.List }
type keyVal struct { key, val int }
func Constructor(capacity int) LRUCache { return LRUCache{ cap: capacity, cache: make(map[int]*list.Element), list: list.New(), } }
func (this *LRUCache) Get(key int) int { if elem, ok := this.cache[key]; ok { this.list.MoveToFront(elem) return elem.Value.(*keyVal).val } return -1 }
func (this *LRUCache) Put(key int, value int) { if elem, ok := this.cache[key]; ok { this.list.MoveToFront(elem) elem.Value.(*keyVal).val = value return } if this.list.Len() >= this.cap { tail := this.list.Back() k := tail.Value.(*keyVal).key this.list.Remove(tail) delete(this.cache, k) } elem := this.list.PushFront(&keyVal{key, value}) this.cache[key] = elem }
|